Spring Security (series) - OAuth Grant Types #4
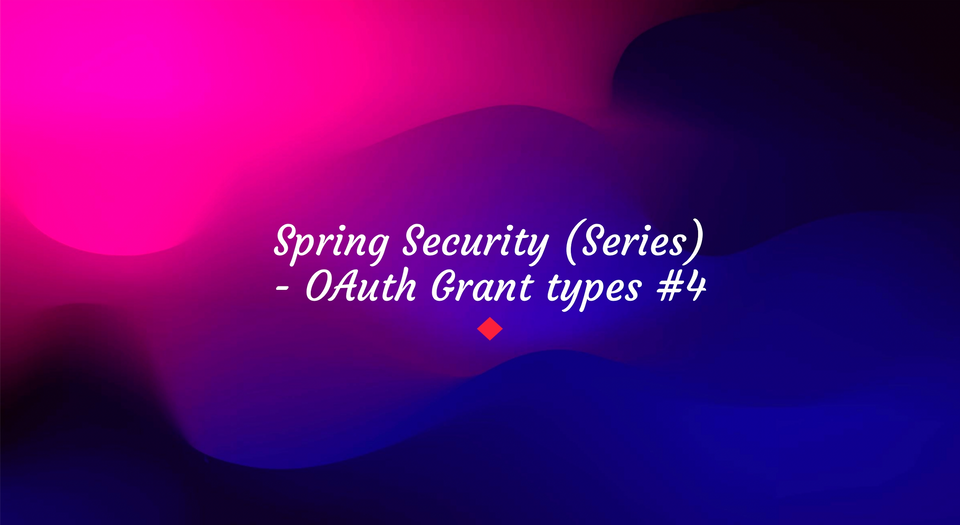
Simple is not always better!
Brief
Let's start with understanding what a Grant Type actually means.
The OAuth grant type defines the flow of the whole OAuth process. It also affects how the client application communicates with the authorization server at each step, including how the access token itself is retrieved.
We'll demonstrate the most common OAuth grant types having as an example a web application made of:
frontend service
- which the users access to interact with resources. It communicates with thebackend service
backend service
- which we'll refer to as the resource serverauthorization server
- which handles the validation of credentials
Implementation
Password (deprecated)
This is a really straight-forward authorization flow.
- The user logs into a form in the
frontend-service
with his username and password. - The
frontend-service
, then forwards the user's information to theauthorization-server
to verify their validity. - If the validation is successful, the
authorization-server
gives back anaccess_token
- The
access_token
will then be added on the request to the resource server.
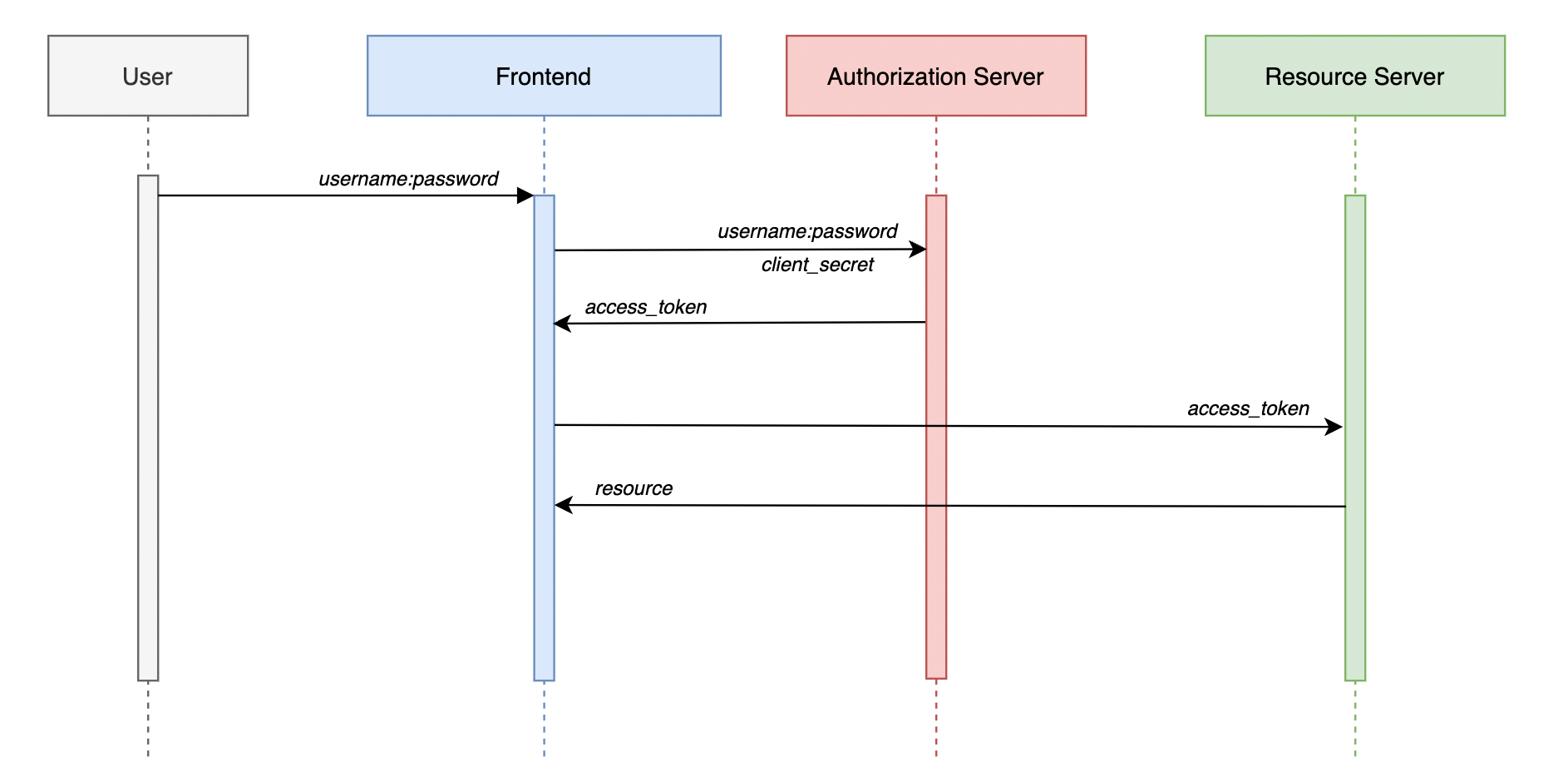
This one became deprecated since we have a lot of points where vulnerabilities can occur.
- multiple components have access to user's credentials (
frontend-service
andauthorization-server
) - encourages phishing attacks
- doesn't support Multi Factor Authentication (MFA) or Single Sign On (SSO)
We can use this grant type for demo purposes since it's really direct, but in a live application it's highly discouraged.
Authorization code
This is the one usually used in production services. It's a bit more complex, but the upside is that it's less vulnerable to malicious attacks.
- The user asks for a resource and is requested to login. Now, the login will not be handled anymore by the
frontend-service
, instead, the user will be redirected to theauthorization-server
. - Here he will use his credentials to login. If the credentials are correct, the
authorization-server
sends a request to thefrontend-service
( on a previously specified endpoint ) with anauthorization_code
. Mind that this is not theaccess_token
recognized by theresource-server
. - In order to get the actual
access_token
, thefrontend-service
needs to send back the receivedauthorization_code
to theauthorization-server
, along with the service's credentials. Theauthorization-server
only responds to known, authenticated services. - If the
authorization_code
is correct and theauthorization-server
recognize thefrontend-service
, only now it returns theaccess_token
. - Finally, with this
access_token
, thefrontend-service
can access theresource-server
.
The advantages of this approach are:
- only one service knows the user's credentials
- the ping-pong with the
authentication_code
keeps us safe from attackers that could fake a redirect url to which theauthorization-server
sends theaccess_token
. By verifying the service's credentials we make sure that our application is the only one that receives to anaccess_token
.
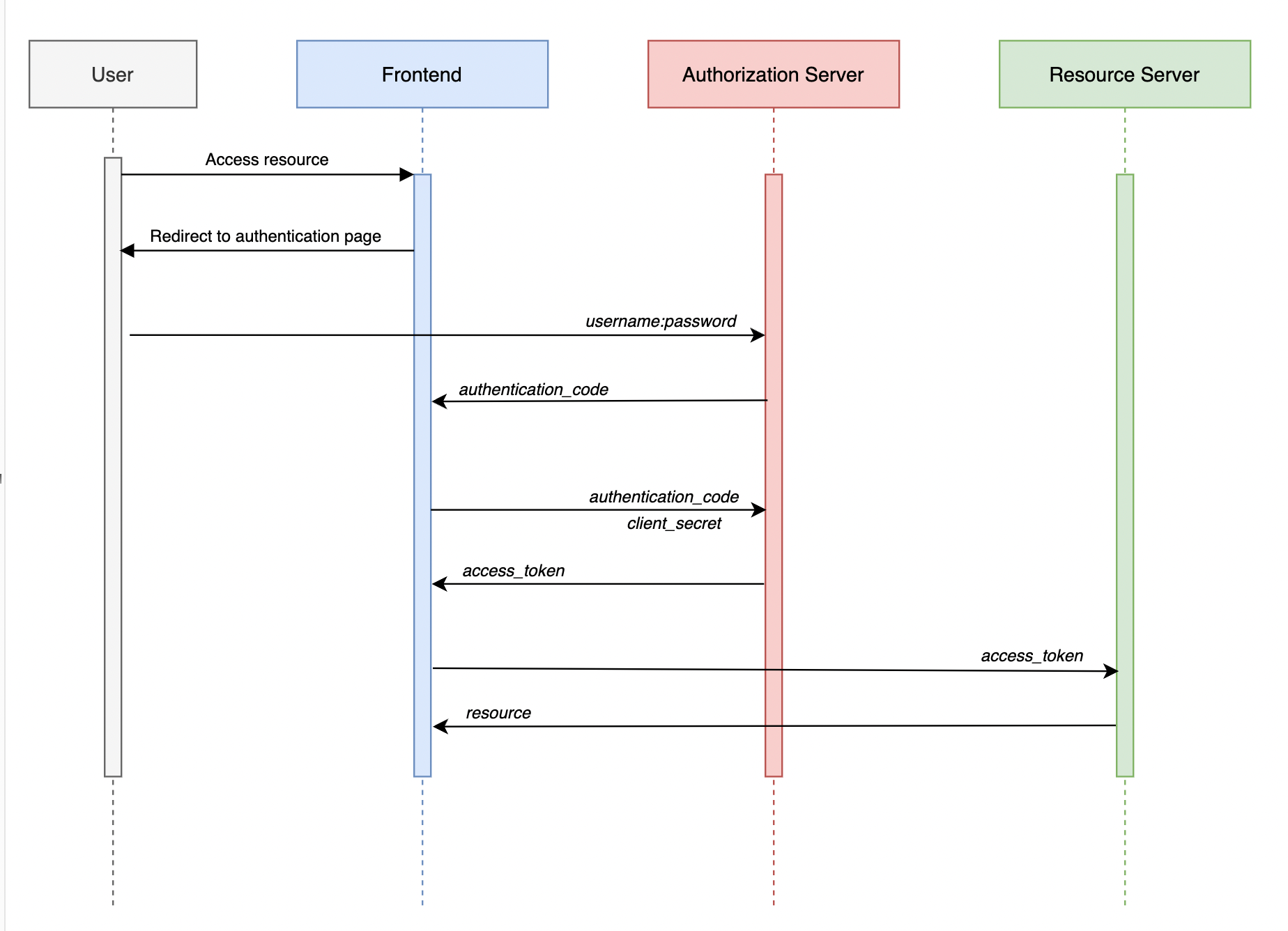
Client credentials
This grant type is used when services in our ecosystem are doing actions without the need for a user intervention. They only rely on service credentials which are internally managed and thus, harder to be accessed by bad intended users.
frontend-service
needs a resource- authenticate on the
authorization-server
using the service's credentials - receive an
access_token
from theauthorization-server
- use the
access_token
to get the resource
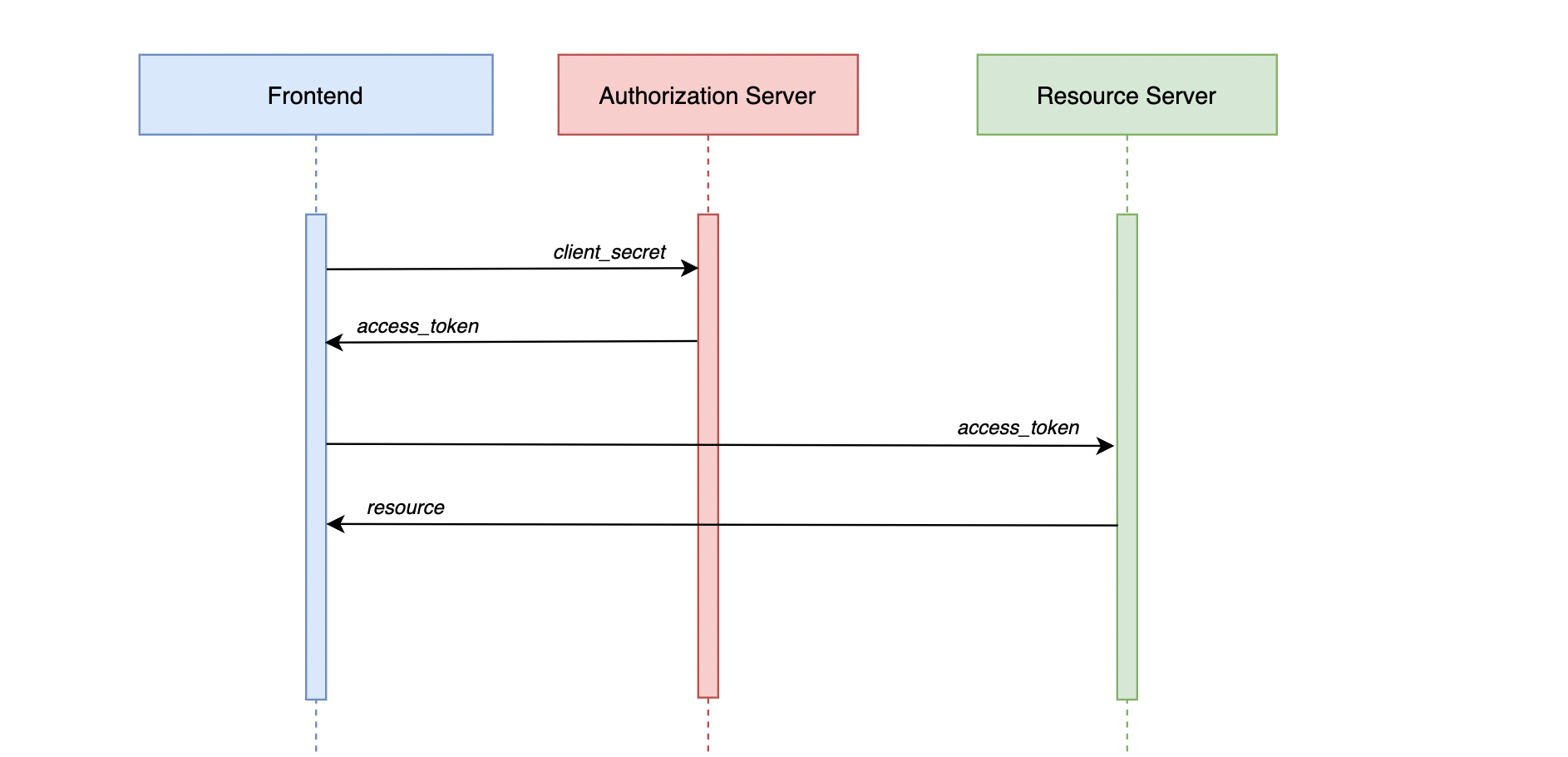
Authorization server
In terms of the authorization-server
component, there are MANY out of the box providers that you can choose from like Okta or Keycloak. You can also implement your own using Spring's Authorization Server implementation and customizing it, the way you want.
Stay tuned! 🚀