Spring Security (Series) - Creating the service #1
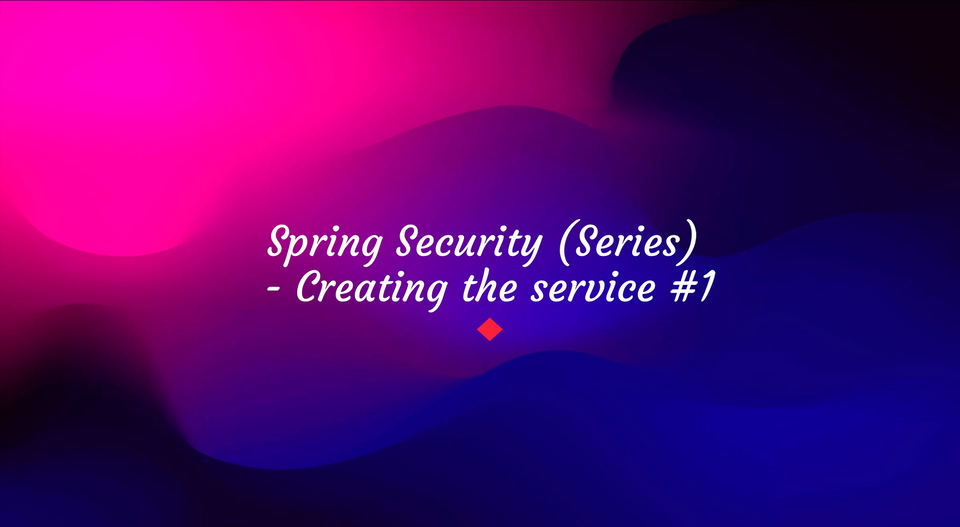
Do you have a lock on your bike but not on your apps? This one is for you.
Brief
Security is a really broad concept, but at its core it relies on two concepts:
- Authentication - the process of recognizing the user and validating his credentials
- Authorization - validation of the actions that user may take
Spring Security is a framework that focuses on providing both authentication and authorization to Java applications. Like all Spring projects, the real power of Spring Security is found in how easily it can be extended to meet custom requirements.
https://spring.io/projects/spring-security
Implementation
Let's start by creating the service.
For this demo we will only require a Spring Boot Web application with a single endpoint returning a dummy value in case of a successful request.
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
@RestController
@RequestMapping("/test")
public class Controller {
@GetMapping
public String test() {
return "Test";
}
}
We can test it using Postman to send a request.
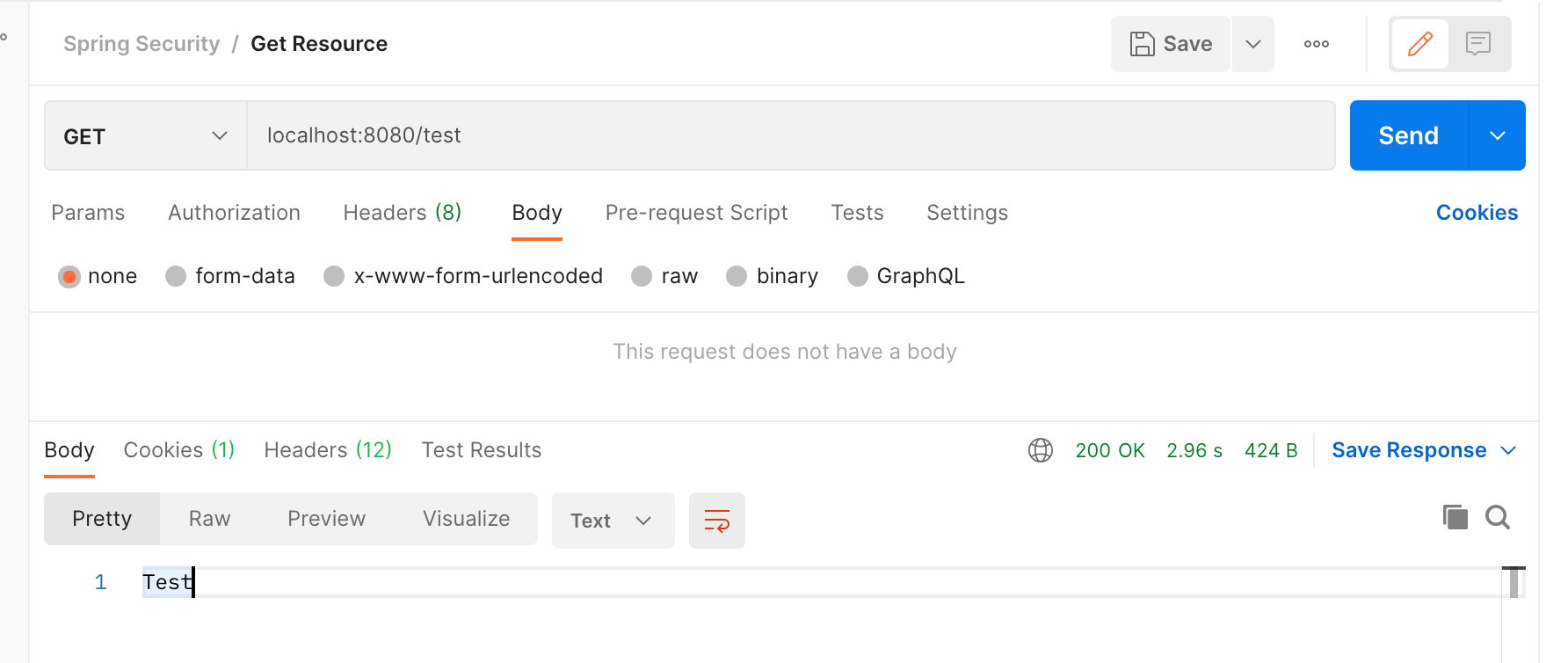
Now, for the part you came here for, let's add the security dependencies.
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-security'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
testImplementation 'org.springframework.security:spring-security-test'
}
Now, all of a sudden, the same request is not working anymore. It's returning 401 Unauthorized
.
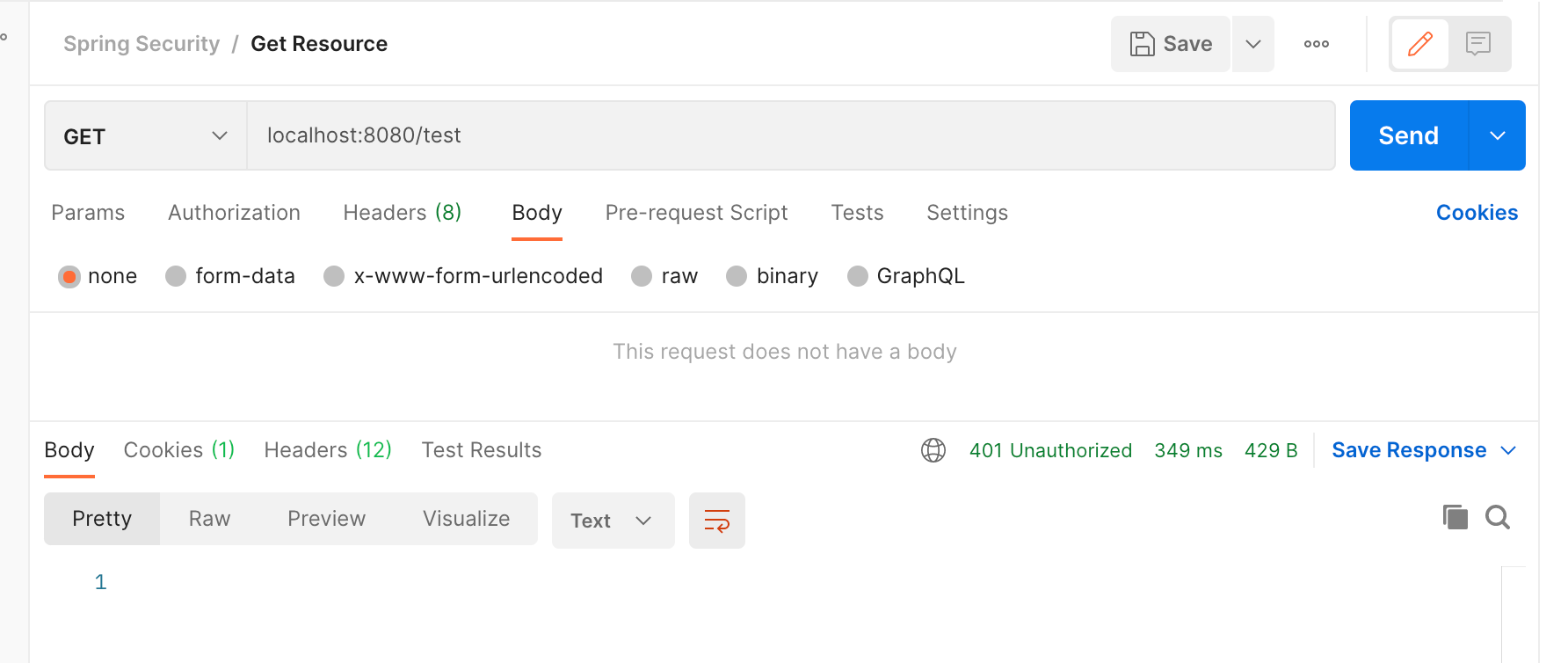
This means that the security module we just added already does its job. And even if we didn't configure anything yet, only by adding the spring-boot-starter-security
dependency, some default configuration was added to our application.
To keep it short (I promise we will get into more details in the next post), we now have a Basic Authentication
put in place with a default user and an auto-generated password. A new one will be generated every time you start the application.
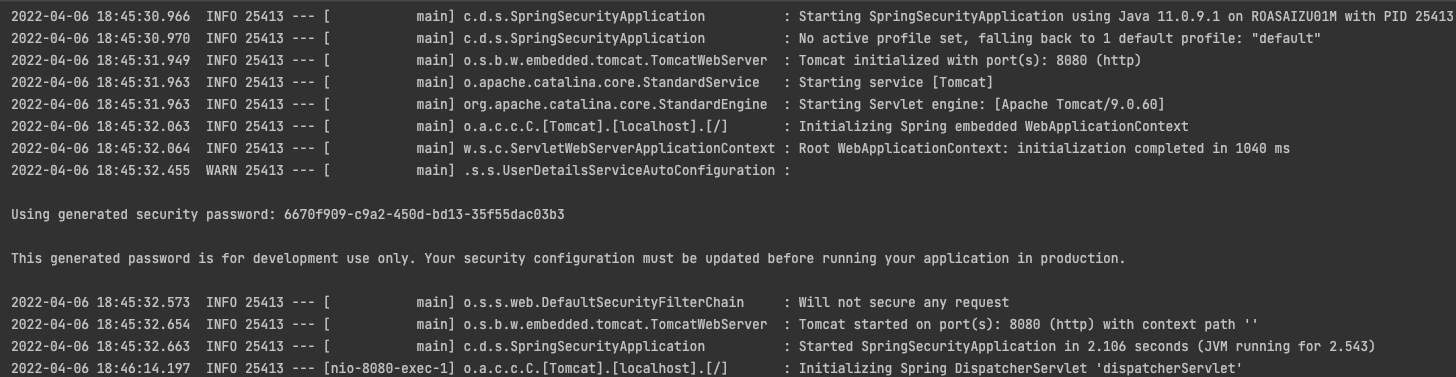
Spring also gives us a nice warning:
"This generated password is for development use only. Your security configuration must be updated before running your application in production."
So what we need to do now, in order to get the same dummy result, is to add the credentials to our request. The default values are:
- user:
user
- password:
{generatedPassword}
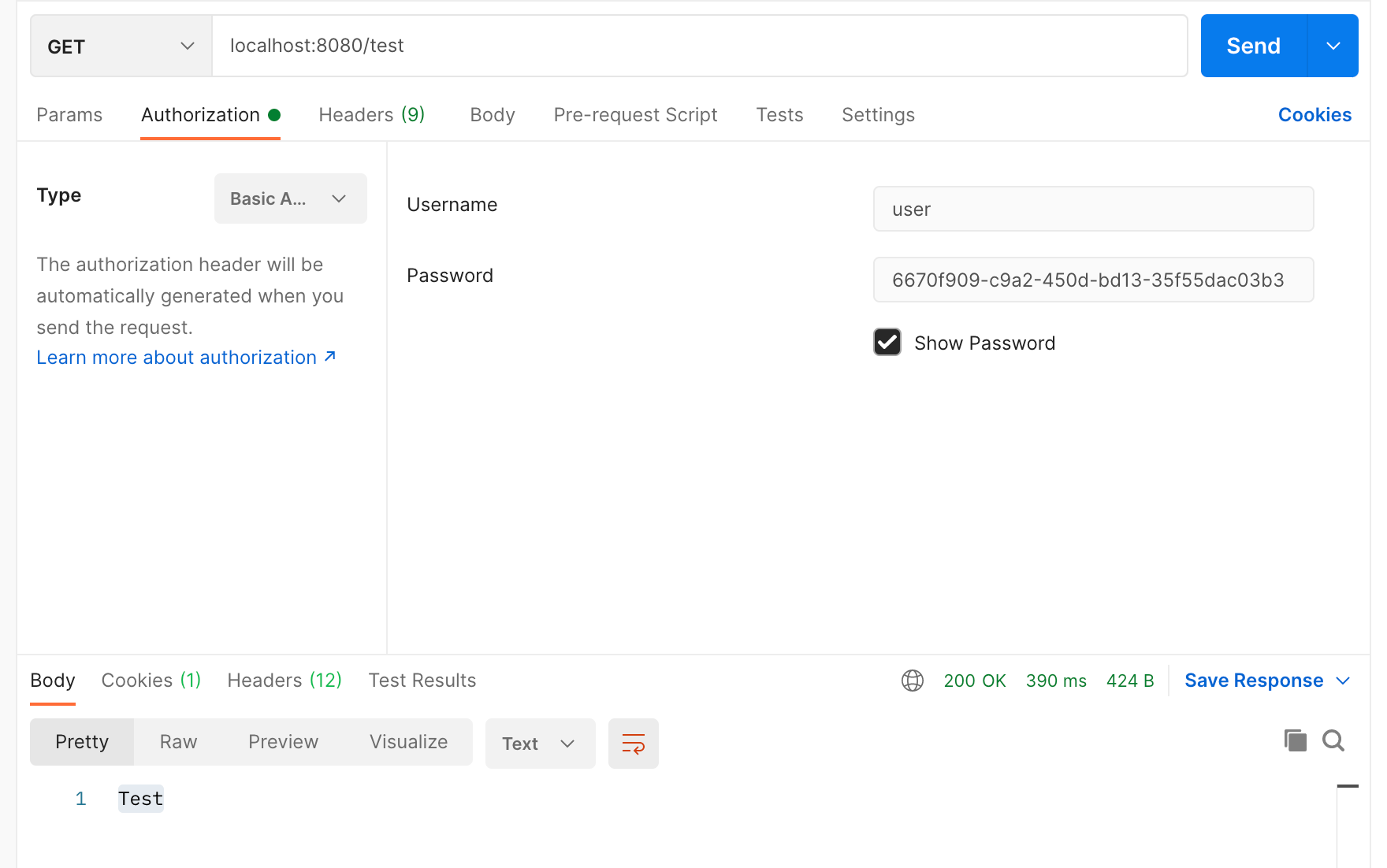
The default credentials can be overriden by adding them in your application.properties
/ application.yml
file.
spring.security.user.name=andrei
spring.security.user.password=password
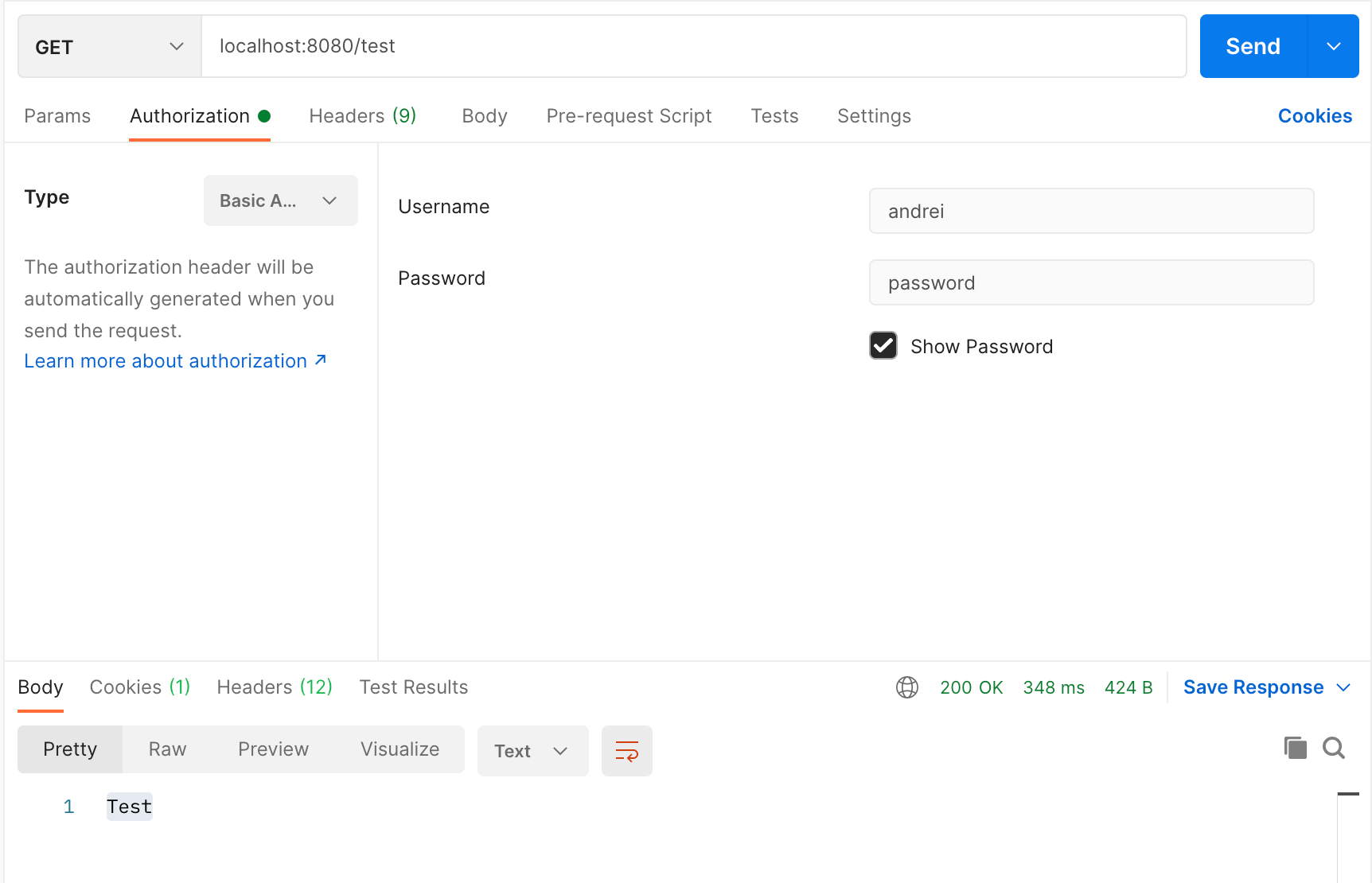
Find the full repository here: https://github.com/andreiszu/spring-security
In the next post, we'll go over Spring Security's flow and how it all works in the background.
Stay tuned! 🚀