Kubernetes ft. Spring Boot (Series) - Creating the services #1
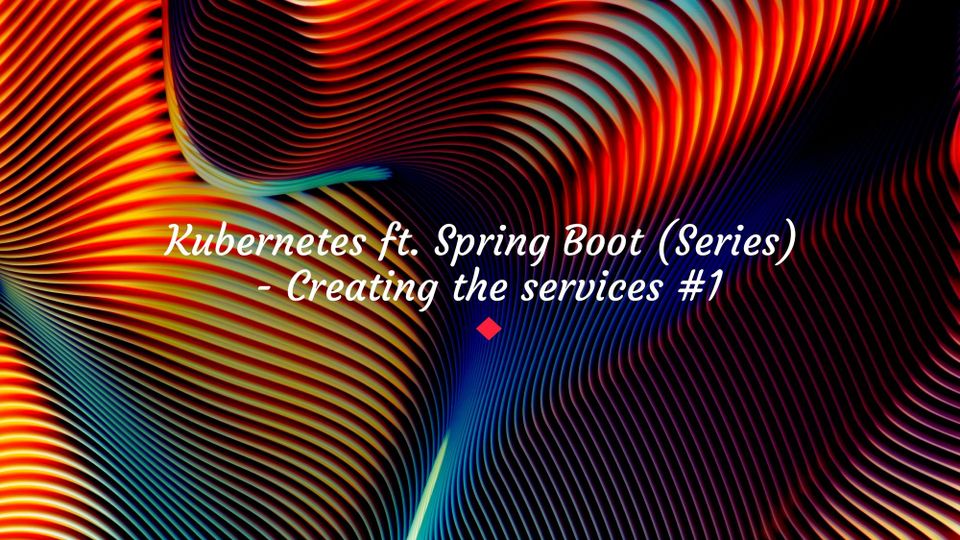
There is life after building the jar
!
Brief
In this series we'll create a Kubernetes environment that will manage 2 simple Spring Boot applications that will communicate with each other through HTTP requests.
We'll have a calling
application and a receiver
application. A client will send a request to the calling
application, this will then send a request to the receiver
application and the response will be returned all the way to the client.

Implementation
For the sake of simplicity, the request from the client
will be passed over to the receiver
service without doing any business logic and will be returned as a response all the way back to the client
.
With the help of Spring Initializr creating the applications is quite straightforward. We'll create two Gradle
projects with Java 11 and we'll only need the Spring Web
dependency added to the projects.
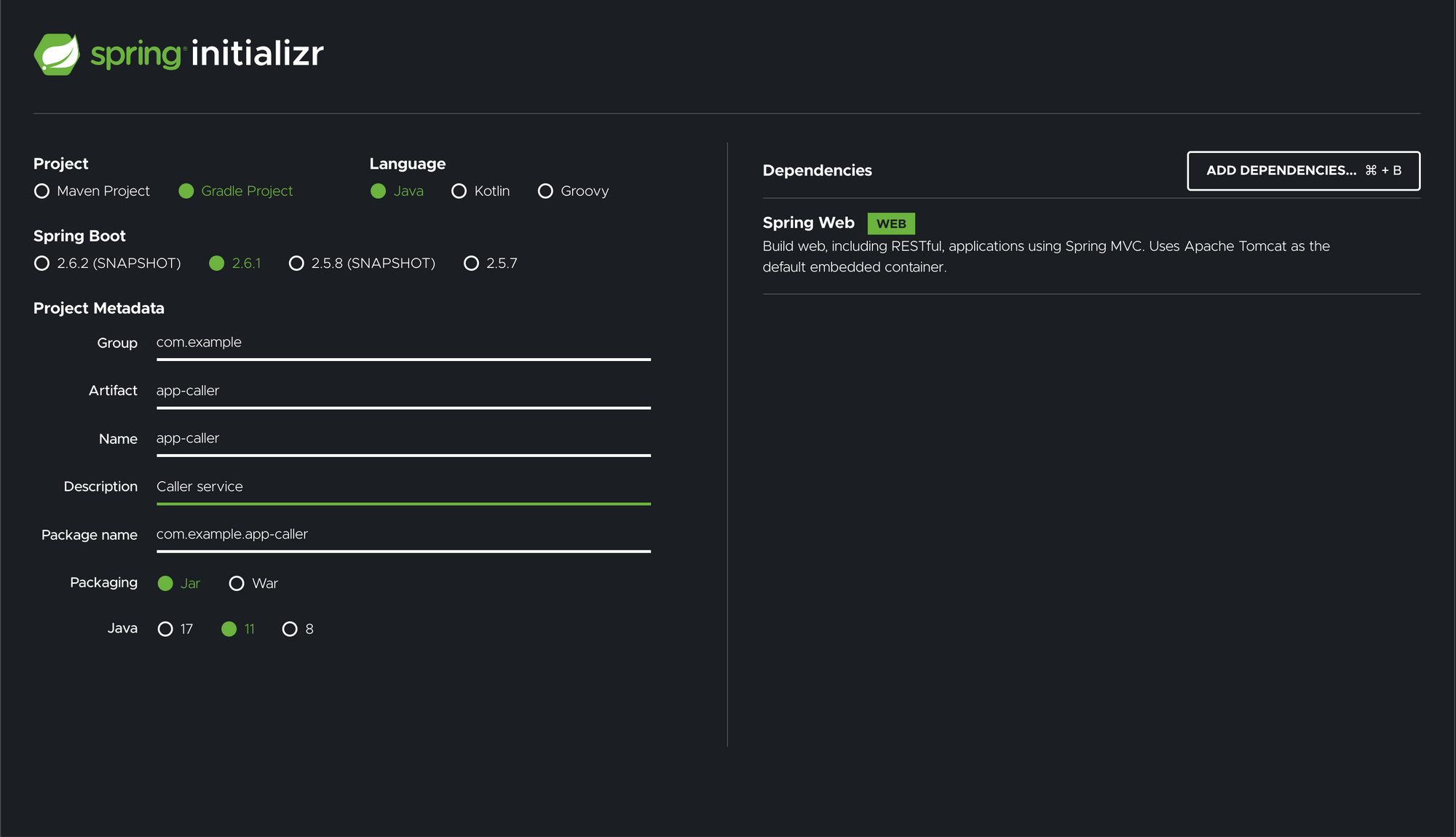
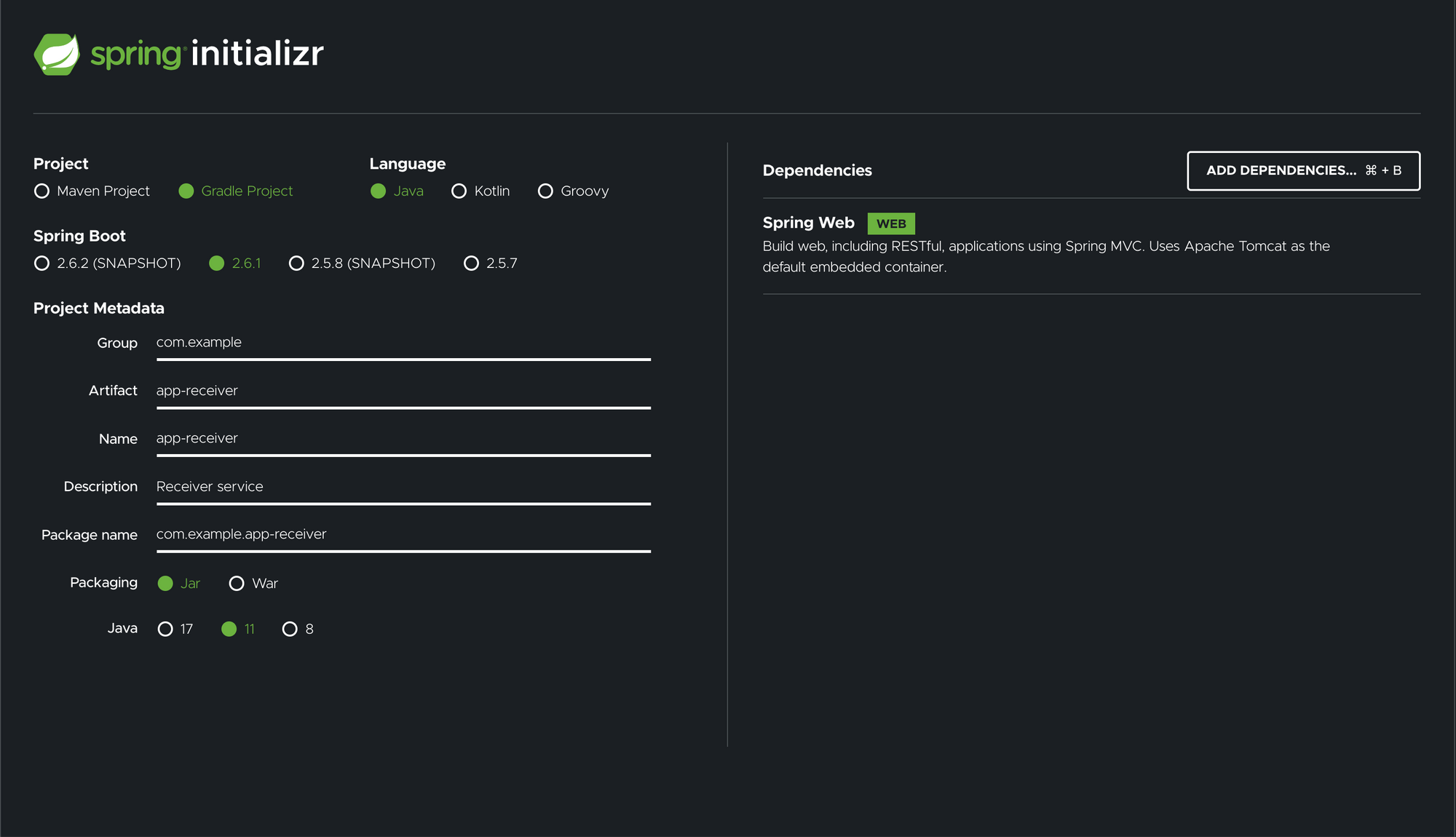
Caller application
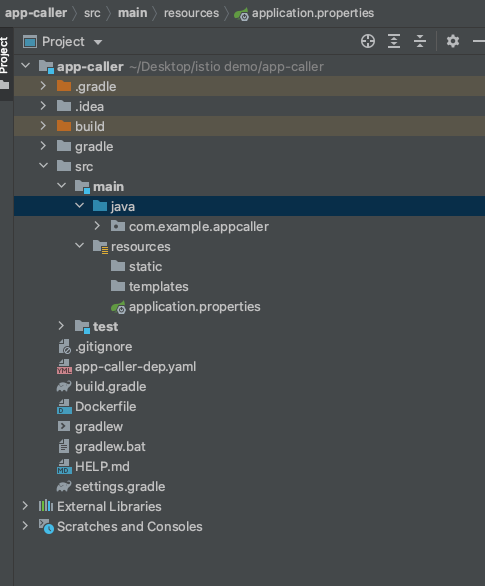
- We'll configure the server to run on port
9991
and have as a context path/caller
. So the client's requests will be sent to:http://localhost:9991/caller
server.port=9991
server.servlet.context-path=/caller
receiver.host=localhost
receiver.port=9992
- Defining the request body DTO
import lombok.Data;
@Data
public class FlowDto {
private String data;
}
- It will also need a REST controller to be able to receive requests from the
client
.
@RestController
@RequiredArgsConstructor
public class Controller {
private final RestClientService restClientService;
@PostMapping("/start")
public ResponseEntity<FlowDto> start(@RequestBody FlowDto flowDto) {
FlowDto response = restClientService.sendStart(flowDto);
return ResponseEntity.ok(response);
}
}
- The service that will actually send the request to the
receiver
application.
@Service
public class RestClientService {
private final RestTemplate restTemplate = new RestTemplate();
@Value("${receiver.host}")
String receiverHost;
@Value("${receiver.port}")
String receiverPort;
public FlowDto sendStart(FlowDto flowDto) {
return restTemplate.postForObject(receiverHost + ":" + receiverPort + "/receiver/start", flowDto, FlowDto.class);
}
}
Receiver application
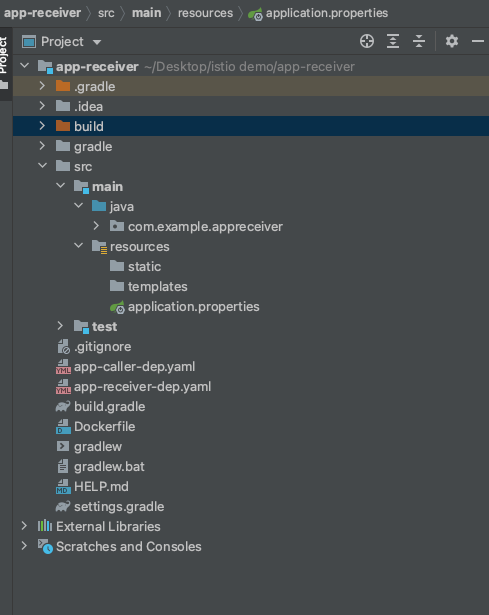
- Server configuration for port and context path
server.port=9992
server.servlet.context-path=/receiver
- Creating the endpoint on the
receiver
application
@RestController
public class Controller {
@PostMapping("/start")
public ResponseEntity<FlowDto> start(@RequestBody FlowDto flowDto) {
return ResponseEntity.ok(flowDto);
}
}
Test
Simulate being the client
by sending a request through Postman to the caller
application.
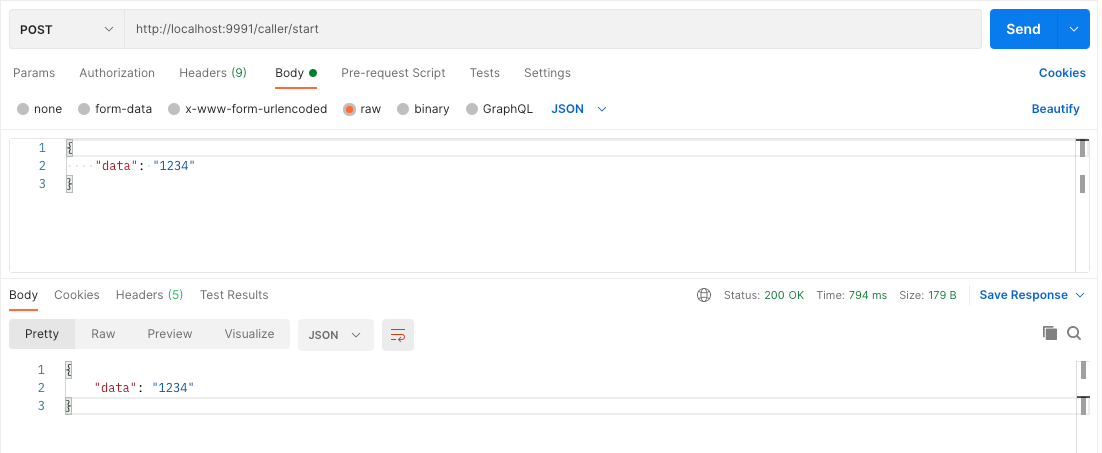
To be continued
In the next post we'll deploy them to a K8s
cluster to see how we can interact with our services in a separate environment rather than localhost
. See you there!
Stay tuned! 🚀