Java Interview Questions (series) - `final` keyword
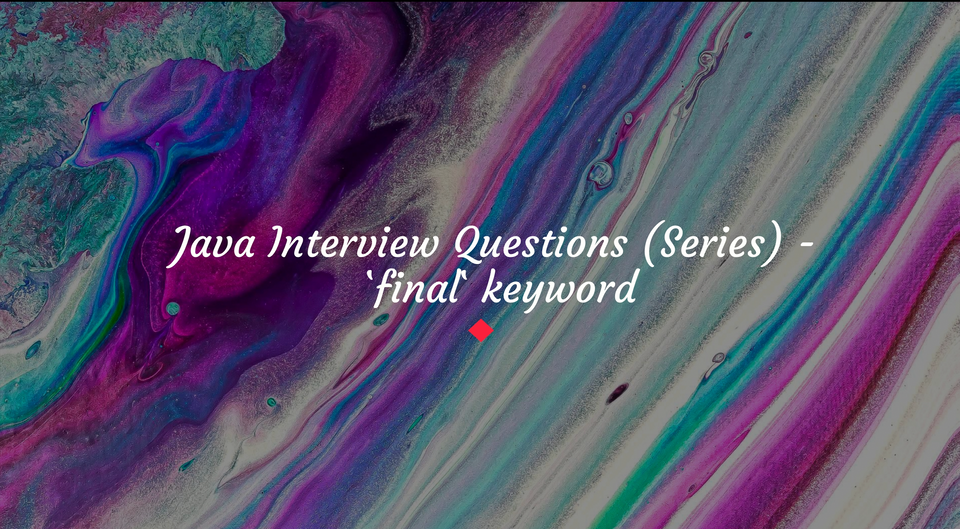
Beware of the F-word! 😈
Brief
The difference between the three Java F-words is one of the interviewers' favourite these days.
They don't have much in common, practically speaking, but they can be easily mistaken.
Let's take them one by one:
1️⃣ Final
- Referring to variables/methods/classes.
2️⃣ Finally
- Find it in the context of exception handling.
3️⃣ Finalize
- Used to release resources used by objects before they're garbage collected.
❗️ We'll split the three into separate posts so you're able to separate them better.
Implementation
1️⃣ Final
Final variable
If you don't want a variable to change its value over the lifecycle of your application, you should add final
in front of it. ⚓️
Example:
final int x = 1; // variable initialization
...
x = 2; // changing its value will result in a compile-time error
❗️ Keep in mind: If a final
variable references an object, it won't keep the object's internals from changing!
final List<Integer> list1 = new ArrayList<>();
list1.add(2); // will work
final List<Integer> list2 = new ArrayList<>();
...
list2 = new ArrayList(); // will not work
Final method
Add the final
keyword in front of a method that you don't want to be overridden by extending classes. 🔒
Example:
public class Animal {
public final int getAge() {
...
}
public void move(){
...
}
}
public class Dog extends Animal {
@Override
public int getAge() { // Won't work! Will result in a compile-time error.
...
}
@Override
public void move() { // Will work!
...
}
}
Final class
Mark a class to be final
when you don't want it to be extended. 🔒
Moreover, it's the first step in creating an immutable class.
public final class Animal {
public final int getAge() {
...
}
public void move(){
...
}
}
public class Dog extends Animal { // Won't work! Will result in a compile-time error.
...
}
Check out the next post where we'll go in-depth about the finally
keyword! 🚀
Stay tuned! 🚀