Java Interview Questions (Series) - String Comparison
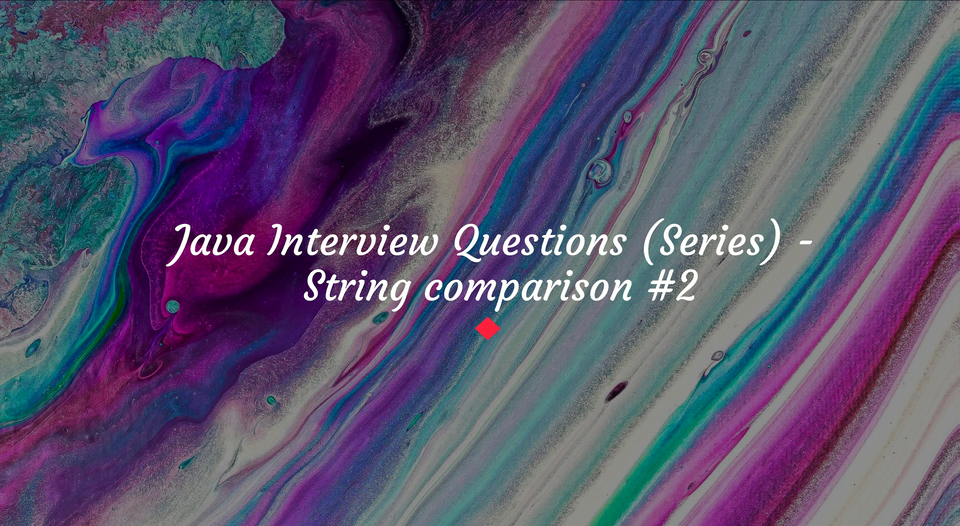
Brief
When asked in an interview how would you go about comparing two Strings
, there should be a few ways coming to your mind.
👀 In this post we'll go over the most common ones:
🔷 ==
🔷 equals(Object x)
🔹 equalsIgnoreCase(String x)
🔷 compareTo(String x)
🔹 compareToIgnoreCase(String x)
Implementation
🔷 ==
Let's take the following Strings
as example:
String x = "1234";
String y = "1234";
String z = new String("1234");
If we were to compare them using the ==
operator, the results will look like this:
x == y -> true
x == z -> false
y == z -> false
❗️ This is because the comparison is done based on the String
references.
If you want to better understand why the second and third lines return false
, check out this post.
🔷 equals(Object x)
Say we have the following case:
String x = "1234";
String y = new String("1234");
Comparing the two Strings
using the equals(Object x)
method, the result will be:
x.equals(y) -> true
❗️ The String
class overrides the equals
method of the parent Object
class as follows:
1️⃣ Checks if the parameter passed to the method is of type String
, if not, it returns false
.
2️⃣ Looks to see if the length of the two Strings
is the same, if not -> false
.
3️⃣ Iterates through the two Strings'
characters and compares them one by one. If any comparison fails, returns false
.
🔹 equalsIgnoreCase(String x)
There may be cases when you don't care about the case of the Strings
. So the comparison result of "Andrei"
and "ANDREI"
should be true
.
❗️ For this case, you can use the equalsIgnoreCase(String x)
method. Behind the scenes, the process looks like this:
1️⃣ Compares the two Strings by reference (==
), if true, it returns the result true
, if not, it goes to the next step.
2️⃣ Looks to see if the length
of the two Strings
is the same, if not, returns false
.
3️⃣ Iterates through the two Strings'
characters and compares them one by one. The difference between this step and the one on the equals
method is that it first applies this ⬇️ instruction on the chars
before comparing them.
Character.toLowerCase(Character.toUpperCase(char))
❗️ You should bear in mind when using equalsIgnoreCase
, that it does not take into consideration the Locale
of the String
and it might result, for some values, in wrong results.
If you know you might be comparing these kind of Strings
it might be a good idea to look over Java's Collator
class which solves this problem.
🔷 compareTo(String x)
The compareTo
method is defined to return:
0
- If theStrings
are equal< 0
- If the firstString
comes before the second in lexicographic order> 0
- If the secondString
comes before the first one in lexicographic order
❗️ The way it works behind the scenes:
1️⃣ It stores the lesser value between the Strings'
lengths in a variable
int len1 = string1.length;
int len2 = string2.length;
int lim = Math.min(len1, len2);
2️⃣ It iterates through the two Strings'
characters until it reaches the lim
index. If two characters on the same position are different, it returns the difference expressed in Integer between the two.
for (int k = 0; k < lim; k++) {
char c1 = string1.charAt(k);
char c2 = string2.charAt(k);
if (c1 != c2) {
return c1 - c2;
}
}
3️⃣ If no characters are different until it reaches the lim
value, the returned result of the method will be the difference between the lengths of the Strings
.
return len1 - len2;
🔷 compareToIgnoreCase(String x)
The same way the equals
method has a cousin 😅 method in equalsIgnoreCase
, compareTo
also has a relative in compareToIgnoreCase
which behaves the same. Moreover, can also take advantage of Collator.
Stay tuned! 🚀