Java Interview Questions (Series) - String Immutability & String Pool
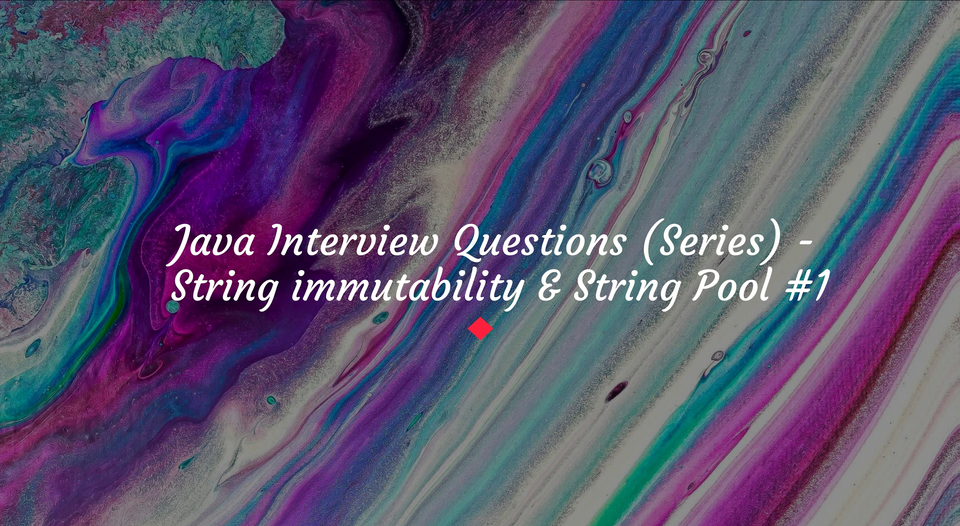
String immutability
From the official Oracle documentation website we find that:
Strings are constant; their values cannot be changed after they are created. (https://docs.oracle.com/javase/7/docs/api/java/lang/String.html)
Hmm.. but if you have ever written a Java application, chances are you tried and successfully changed the value of a String variable.
But now, seeing that description, how was that even possible?
Did Java lie to us?? 😅
🔵 Let's take a step back and see the ways of how we can instantiate a new String.
String text = "test";
String text = new String("test")
;
The result of these two is the same, we get a variable named text
of type String with the value of test
.
The main difference of the two is the way the JVM initialises the variable.
Java String Pool
The Java String Pool
is the memory area in which String literals are stored by the JVM.
Let's take some examples and see what is going on behind the scenes:
🔹1. String text = "Mark";
1. Looks for a "Mark" value in the String Pool.
2. Doesn't find it and adds it to the Pool.
3. Assigns the String reference to the variable.
🔹2. text = "Mark Twain";
1. Looks for a "Mark Twain" value in the String Pool.
2. Doesn't find it and adds it to the Pool.
3. Reassigns the String reference to the variable.
🔹3. String stringObject = new String("new Object");
1. Creates a String in the Heap space.
2. Assigns the String reference to the variable.
🔹4. stringObject = stringObject.intern();
1. Adds the String from the Heap space to the Java String Pool.
2. Reassigns the String reference to the variable.
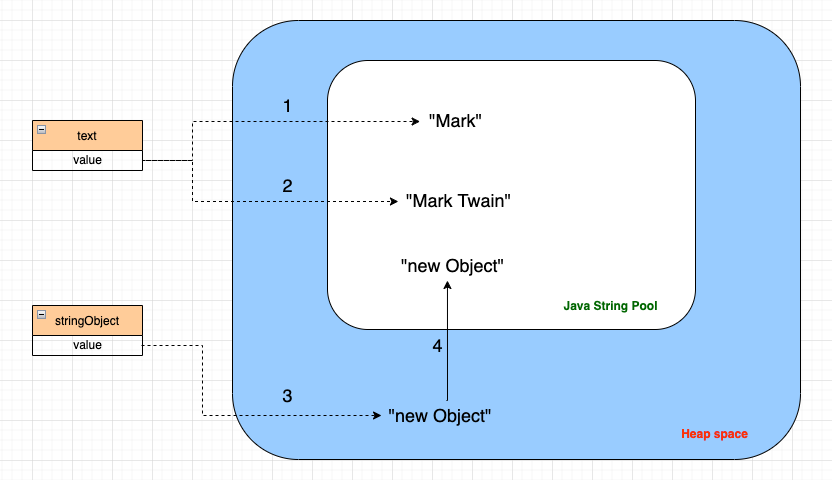
⚓️ Because Strings are immutable, the JVM is able to optimise the amount of memory allocated by storing each literal String
once in the pool.
Since Java 7 , the Java String Pool
is stored in the Heap
space, which is garbage collected by the JVM. That means unreferenced Strings will be removed from the pool, thereby releasing memory.
Stay tuned! 🚀