Java Interview Questions (series) - Lambda expressions
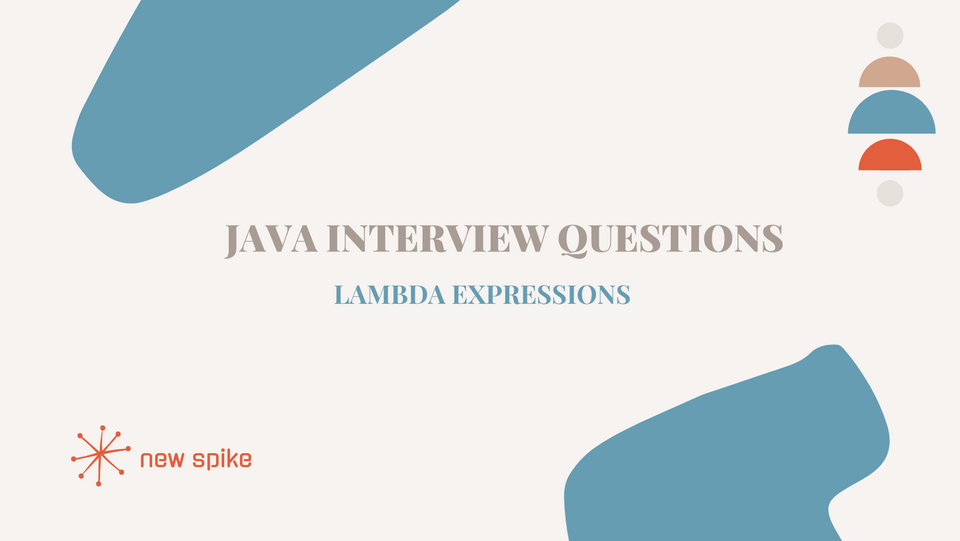
Less is more when it comes to Java! 💯
Brief
Following up on the previous post, today we'll cover:
🔸 What is a lambda expression
?
🔸 The relation between lambda
and Functional Interfaces
🔸 The relation between lambda
and Streams
🔸 Implementation examples.
Implementation
🔵 The lambda
expression is used to provide the implementation of a functional interface.
The main advantage of it is that it reduces a lot of code needed to provide the implementation.
Let's see how you can do it! 👀
1️⃣ First, you'll need a functional interface. If you want a better understanding of what it is and how it works, check out this post!
@FunctionalInterface
public interface Accumulator {
int add(int x, int y);
}
2️⃣ Implement the interface.
Note
Before Java 8, if you didn't want to have a separate class implementing your interface, you could do it using an anonymous class.
int x = 10;
int y = 15;
// anonymous class
Accumulator accumulator = new Accumulator() {
@Override
public int add(int x, int y) {
return x + y;
}
};
int result = accumulator.add(x, y); // result = 35
🚀 From Java 8
forward you can implement the functional interface in a more quicker and readable way using a lambda expression.
int x = 15;
int y = 20;
// lambda expression
Accumulator accumulator = (x1, y1) -> x1 + y1; // implementation of the interface method
int result = accumulator.add(x,y); // result = 35
Another important difference between a lambda
and an anonymous class
is that, for the latter, the Java compiler actually creates a separate .class
file just how it would do for a usual class implementing the interface.
Whereas the lambda
expression is treated like a simple method.
🤝 You will also find lambda expressions working hand in hand with Streams
.
You can do changes on items in the stream, you can filter out some of it and a lot more.
🔹 Let me give you an example.
List<Integer> inputList = new ArrayList<>();
inputList.add(1);
inputList.add(2);
inputList.add(3);
inputList.add(4);
inputList.add(5);
List<Integer> filteredList = inputList.stream()
.filter(item -> item % 2 == 0) // lambda expression returning true for odd items
.collect(Collectors.toList());
// filteredList = { 2, 4 }
🔹 Also, the lambda block doesn't have to be only a single line long.
List<Integer> inputList = new ArrayList<>();
inputList.add(1);
inputList.add(2);
inputList.add(3);
inputList.add(4);
inputList.add(5);
inputList.forEach(item -> {
item++;
System.out.println("Updated item: " + item);
});
// Result:
// Updated item2
// Updated item3
// Updated item4
// Updated item5
// Updated item6
👍 The rule of thumb, though, is that if you need to have multiple lines in a lambda expression, extract it in a private method.
public class Calculator {
public static void calculate() {
List<Integer> inputList = new ArrayList<>();
inputList.add(1);
inputList.add(2);
inputList.add(3);
inputList.add(4);
inputList.add(5);
inputList.forEach(item -> extracted(item));
}
private static void extracted(Integer item) {
item++;
System.out.println("Updated item: " + item);
}
}
🧠 Or even better, based on the method signature, the compiler can figure out what you are trying to do.
That means you can call the method just by passing the class name along the method name.
public class Calculator {
public static void calculate() {
List<Integer> inputList = new ArrayList<>();
inputList.add(1);
inputList.add(2);
inputList.add(3);
inputList.add(4);
inputList.add(5);
inputList.forEach(Calculator::extracted); // lambda using method reference
}
private static void extracted(Integer item) {
item++;
System.out.println("Updated item: " + item);
}
}