Java Interview Questions (Series) - Functional Interface
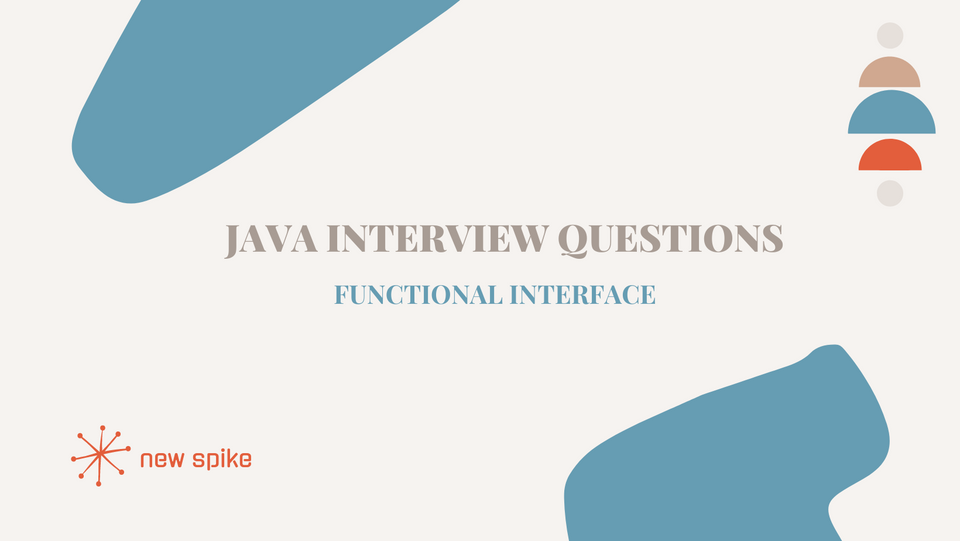
The devil is always in the details! 😈
Brief
If you are to join a new project that's using at least Java 1.8 ( if not, you should consider looking for another project), you should be able to explain and what is a FunctionalInterface
and what it is made of.
Implementation
Let's get into it! 🚀
A FunctionalInterface
in Java is an interface having only one abstract method.
That's it!
@FunctionalInterface
public interface Flyable {
void fly();
}
❗️ Keep in mind that the @FunctionalInterface
annotation is not mandatory, but it's a good practice to add it for it's validation purpose.
If you have an interface annotated with @FunctionalInterface
and you try to add a second abstract method, you will get a nice compile-time error telling you that:
Multiple non-overriding abstract methods found in: YourFunctionalInterface.java
.
🤨 Wait a minute, the compiler is not complaining that there are multiple abstract methods in your interface, but that they are non-overriding
.
❓ Hmm, does it mean that we can have multiple abstract but overriding methods in our functional interface?
The answer is yes! ✅
Long story short
Every Java interface contains abstract methods with the same signature from Object class for equals
, toString
and hashCode
, methods.
public interface YourInterface {
String toString(); // this method is already there but you can still explicitly declare it
}
For this reason, they are not taken into account when validating a FunctionalInterface
.
🧩 If this part was a bit confusing, it's totally fine, let's summarize it:
A FunctionalInterface
can contain:
🔸 one abstract non-overriding method
🔸 any number of abstract overriding methods // those from Object
🔸 any number of default methods
🔸 any number of static methods.
Full example
@FunctionalInterface
public interface Flyable {
void fly();
String toString(); // overriding method from Object class
default void defaultMethod() {
System.out.print("Log from default method!");
}
static void staticMethod() {
System.out.print("Log from static method!");
}
}
Bonus
If you're not familiar with default
and static
methods in a Java interface and what they are used for, keep a close eye on your email inbox.
I am working on a Java Updates Series
where I describe the new features that come with each version of Java starting with 1.8.
These ones will be present in the very first post!