Java Interview Questions (Series) - StringBuffer vs StringBuilder
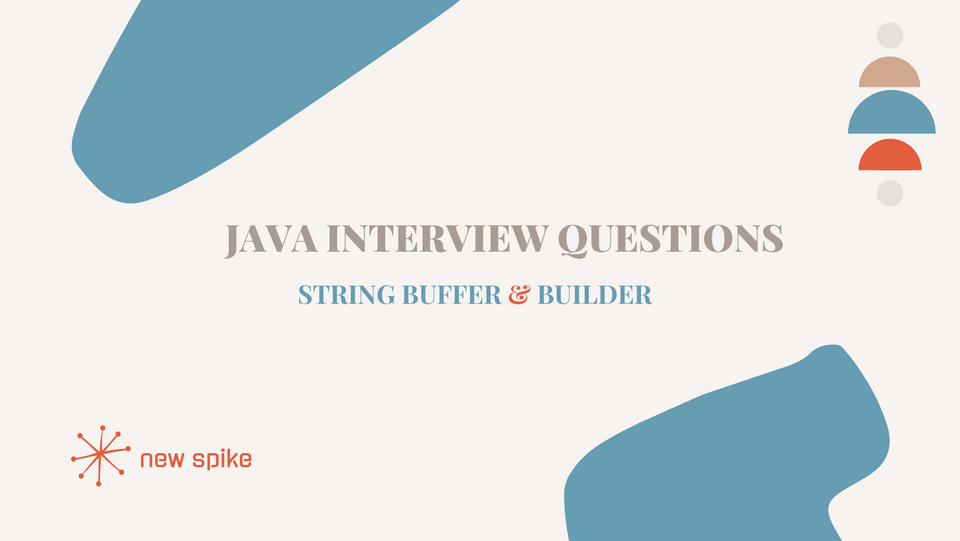
Change is inevitable except from a vending machine. - Robert C. Gallagher
Brief
If you are a Java developer you definitely changed a String's
value before.
❓ But did you do it correctly❓
We found out in this previous post, that the String
class is immutable in Java and saw what actually happens behind the scenes.
❓ In this case, how should we go about String
alteration?
After asking themselves this question, the Java creators came up with a solution.
Actually, they came up with two ways for modifying a String
.
1️⃣ StringBuffer
which is with us since the first version of Java
2️⃣ StringBuilder
which came around in Java 1.5
Implementation
StringBuffer
🧩 It is a class that can hold a sequence of characters.
All its public methods are synchronized
that means it's thread-safe.
🚦 Let's see some practical examples of using StringBuffer
:
🔸 initialize
StringBuffer sb1 = new StringBuffer();
StringBuffer sb2 = new StringBuffer("Init"); // initializing with value
🔸 append values at the end
StringBuffer sb = new StringBuffer("1234");
sb.append(5); // append "5" at the end
String value = sb.toString(); // "12345"
append
method is overloaded to be able to accept a lot of types of input ( int
, float
, char
, etc.)
🔸 insert values at a certain position
StringBuffer sb = new StringBuffer("1234");
sb.insert(2,5); // insert "5" at index 2
String value = sb.toString(); // "12543"
insert
method is also overloaded to be able to accept different types of input ( int
, float
, char
, etc.)
🔸 remove values
StringBuffer sb = new StringBuffer("1234");
sb.delete(1,3); // specify the index range of chars to be removed
String value = sb.toString(); // 14
sb.deleteCharAt(0); // specify the index of the char to be removed
String newValue = sb.toString(); // 4
StringBuilder
🧩 It's also a class capable of holding and manipulating a character sequence.
It is really similar to StringBuffer
in terms of methods, only lacking some that are already found at String
class level, like length()
, substring()
, etc.
❗️ The main difference between them is that the methods on StringBuilder
are not synchronized!
This means that StringBuilder
is not safe to be used in a multi-threaded application.
I'll do a post on multi-threaded Java applications where I'll go into a lot more details about the dragons that sleep in there.
🏁 On the other hand, the advantage of the methods not being synchronized
is that they are a lot faster.
Because of that, if you don't care about thread-safety, StringBuilder
is the way to go for your String
manipulations.
Bonus
Going back, you probably used neither of the two.
You can alter the String
using the +
operator like this:
String address = "My " + "Street";
And it is a totally fine way of doing it. But what is going on in there?
The +
operator between two Strings
is transformed by the compiler in:
String address = (new StringBuilder()).append("My ").append(" Street").toString();
That means, even if that +
is more straight-forward you might have problems with multiple threads doing it.
Stay tuned! 🚀