Spring Security (series) - GitHub SSO #5
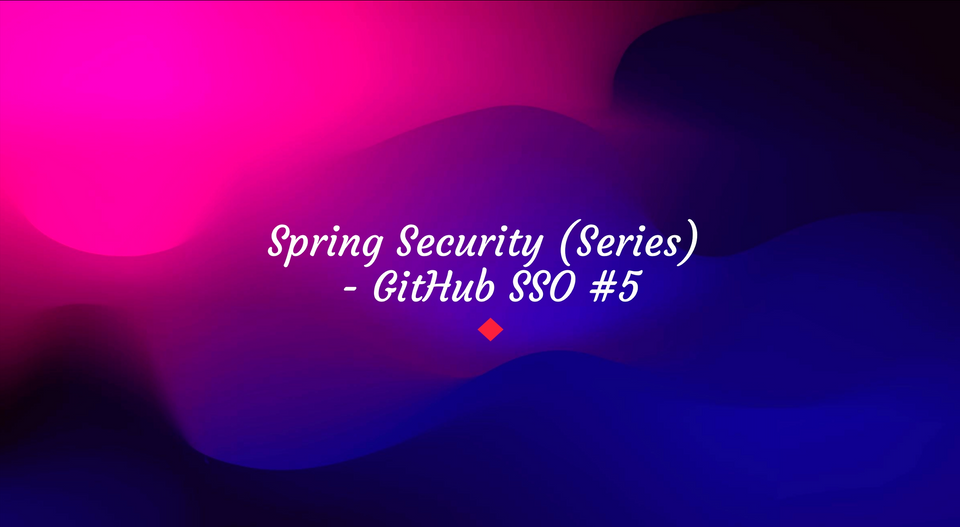
Let GitHub handle the headache of your user management.
Brief
I always wanted to know how that magic 'Sign in using your GitHub account' works. After some research I found that it's actually not complicated at all.
Implementation
First, you need to go to GitHub and register your application at https://github.com/settings/developers
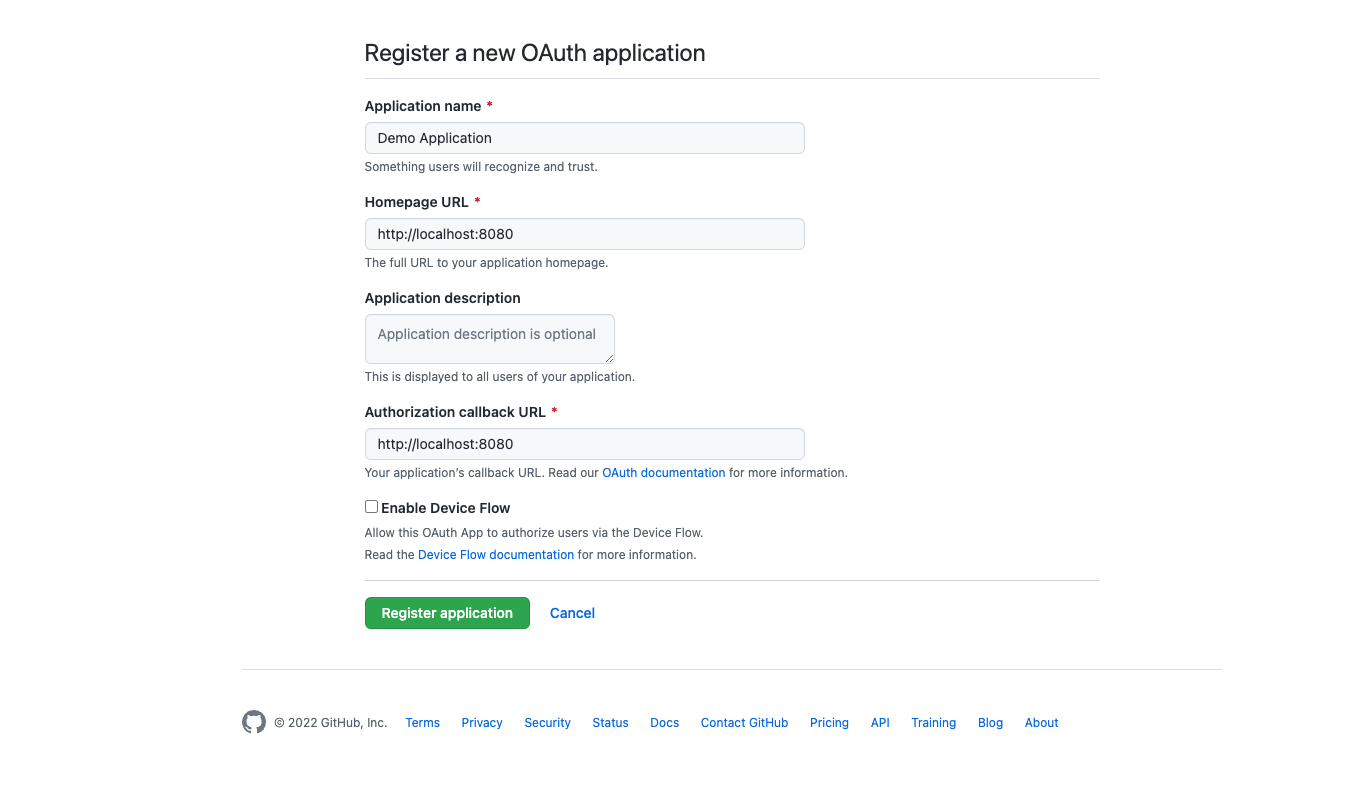
You will need to generate a clientSecret
, which you will need along with the clientId
in the security configuration.
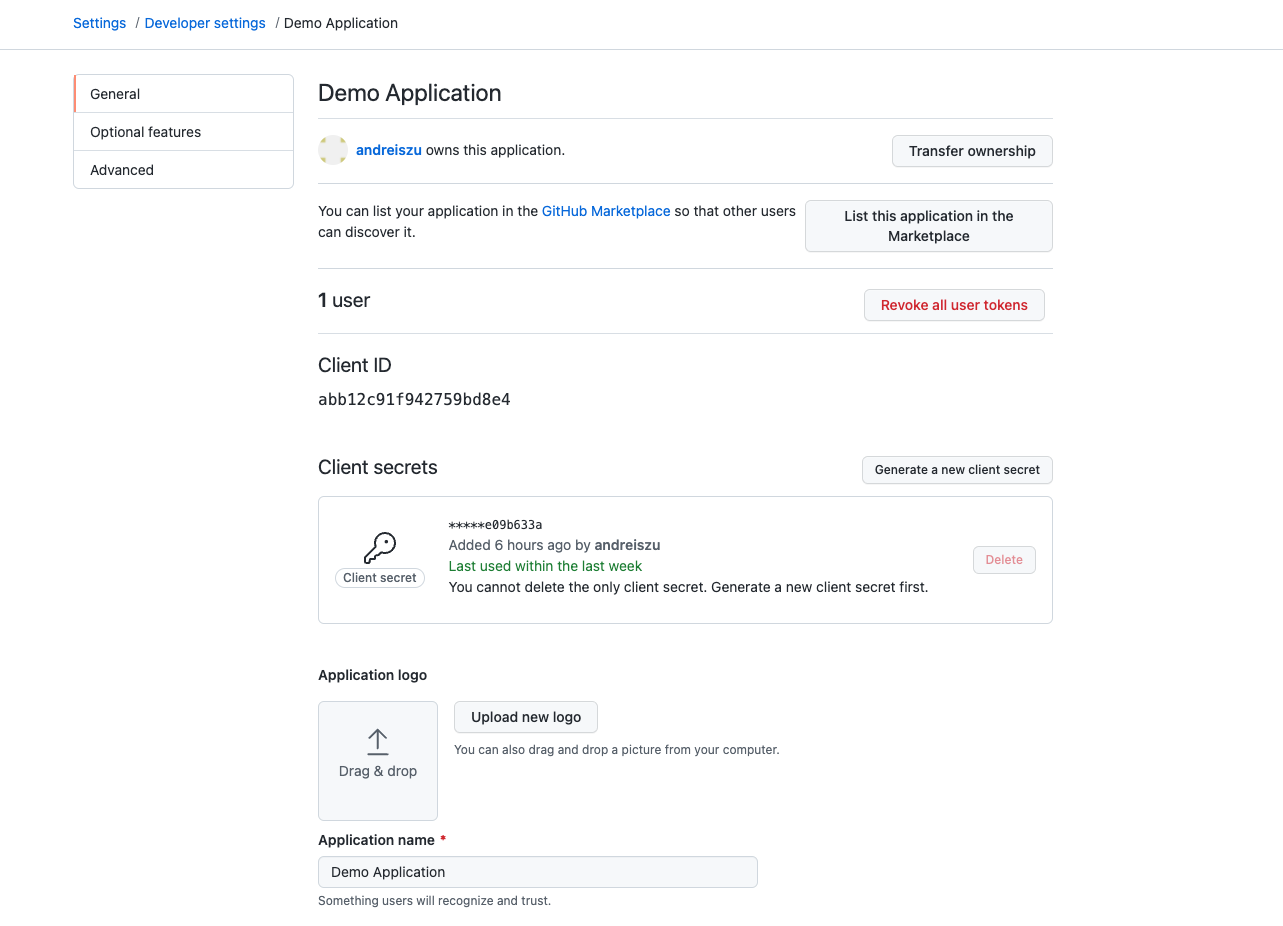
Let's create the simplest application possible.
We'll have a controller returning a static html page. The controller should only be accessed by logged in users.
@Controller
@RequestMapping("/hello")
public class HelloController {
@GetMapping
public String hello() {
return "index.html";
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Demo application</title>
</head>
<body>
<h1>GitHub sign in!</h1>
</body>
</html>
We need to add the oauth2-client
dependency in our build.gradle
file.
---
implementation 'org.springframework.boot:spring-boot-starter-oauth2-client'
---
The security configuration is really straight-forward.
- As always, we'll extend
WebSecurityConfigurerAdapter
and override theconfigure(HttpSecurity http)
. - Add a
ClientRegistration
bean in Spring'sClientRegistrationRepository
containing details about reaching GitHub.
@Configuration
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.oauth2Login();
http.authorizeRequests().anyRequest().authenticated();
}
@Bean
public ClientRegistrationRepository clientRegistrationRepository() {
return new InMemoryClientRegistrationRepository(gitHubClient());
}
private ClientRegistration gitHubClient() {
return CommonOAuth2Provider.GITHUB.getBuilder("github")
.clientId("abb12c91f942759bd8e4")
.clientSecret("674bfb0041309ecd38a486b90b69928fe09b633a")
.build();
}
}
You should never store your credentials directly in code, they should be injected from a Vault or some other secret storage implementation.
You can also create your custom ClientRegistration
where you would need to add details about the authorization server. See below details about the Github client.
GITHUB {
@Override
public Builder getBuilder(String registrationId) {
ClientRegistration.Builder builder = getBuilder(registrationId,
ClientAuthenticationMethod.BASIC, DEFAULT_REDIRECT_URL);
builder.scope("read:user");
builder.authorizationUri("https://github.com/login/oauth/authorize");
builder.tokenUri("https://github.com/login/oauth/access_token");
builder.userInfoUri("https://api.github.com/user");
builder.userNameAttributeName("id");
builder.clientName("GitHub");
return builder;
}
}
Aaand that's all the code you need. Let's see it live.
If you now go into your browser at localhost:8080/hello
, you should be redirected to the GitHub login form. After providing correct credentials of your account, you will be prompted to authorize the browser to access your backend application.
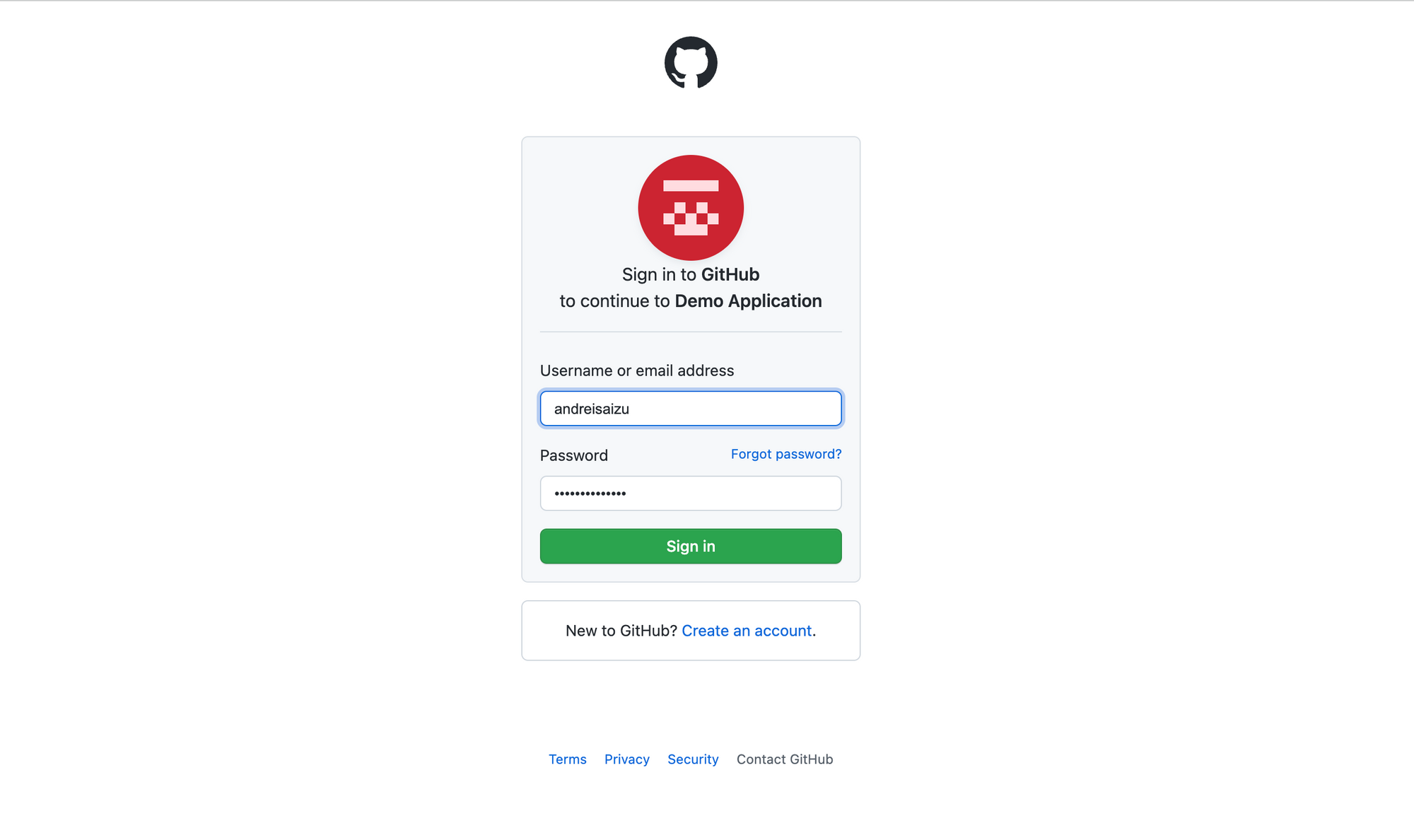
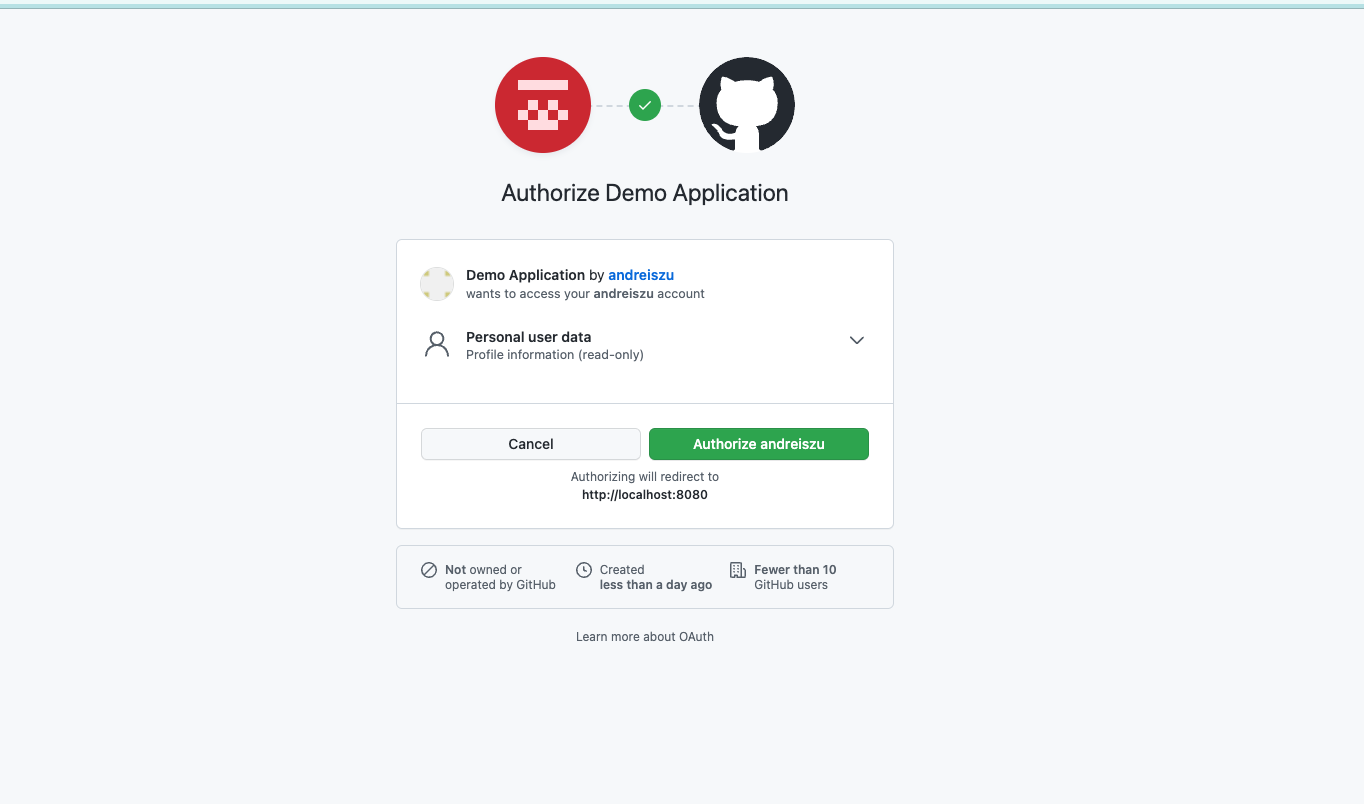
Finally, you should see the beautifully designed web page standing behind your controller.
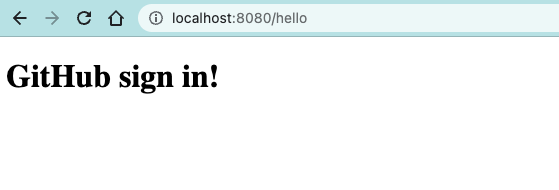
Like always, checkout the full code here.
Stay tuned! 🚀