OpenAPI & Postman - MockServer
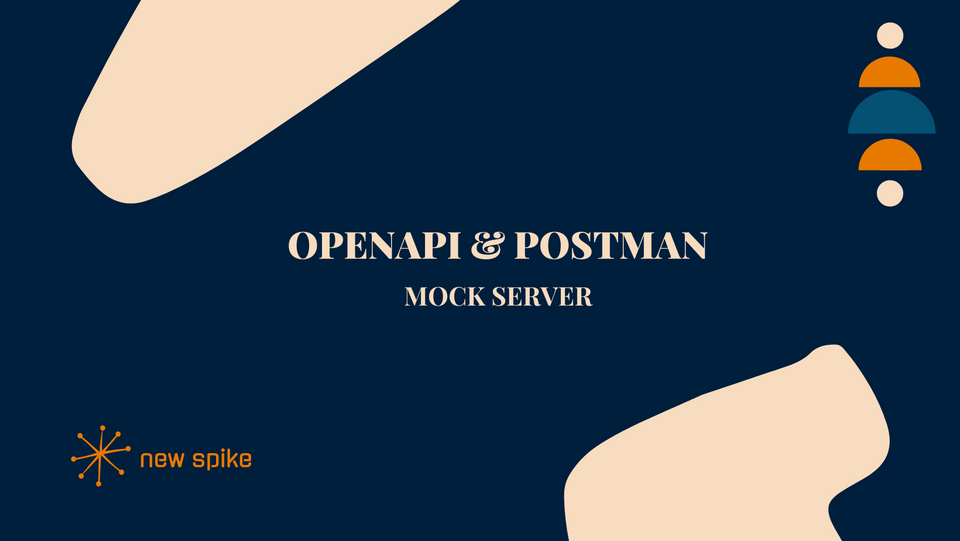
Integration testing is a must❗️
Intro
When you're working on a frontend - backend
project where the frontend application serves some resources brought in by the backend application, you definitely ran into the issue of frontend team
not really being able to work until the backend endpoints are ready. 🤝
For this reason, the API First
approach came about. 💯
It states that you should first define the contract between client and server before starting working on implementations.
This way, the two teams can work in parallel as long as they respect that contract.
The backend team
knows how it should retrieve the data, and the frontend team
knows what to expect as a response to requests. 🚀
Testing
🔵 Backend
In order to do integration testing on the backend side, you can write code that simulates your endpoints being called and/or
( I prefer and
) you use Postman to send actual HTTP requests to your BE application.
🔵 Frontend
But can Postman help the FE team too?
The answer is yes!
Postman has the concept of Mock Servers
.
First, you need to create some requests calling the BE application.
For each of them, you add some Examples
where you specify a mock response for your endpoints.
Then, based on this collection of requests Postman can generate a Mock Server
for you.
🔵 Implementation
1️⃣ Generate OpenAPI schema. ( https://app.swaggerhub.com/home)
openapi: 3.0.0
info:
description: |
This is a sample Petstore server. You can find
out more about Swagger at
[http://swagger.io](http://swagger.io) or on
[irc.freenode.net, #swagger](http://swagger.io/irc/).
version: "1.0.0"
title: Swagger Petstore
servers:
- url: 'https://petstore.swagger.io/v2'
tags:
- name: pet
description: Everything about your Pets
paths:
/pet:
post:
tags:
- pet
summary: Add a new pet to the store
operationId: addPet
responses:
'405':
description: Invalid input
requestBody:
$ref: '#/components/requestBodies/Pet'
put:
tags:
- pet
summary: Update an existing pet
operationId: updatePet
responses:
'400':
description: Invalid ID supplied
'404':
description: Pet not found
'405':
description: Validation exception
requestBody:
$ref: '#/components/requestBodies/Pet'
'/pet/{petId}':
get:
tags:
- pet
summary: Find pet by ID
description: Returns a single pet
operationId: getPetById
parameters:
- name: petId
in: path
description: ID of pet to return
required: true
schema:
type: integer
format: int64
responses:
'200':
description: successful operation
content:
application/json:
schema:
$ref: '#/components/schemas/Pet'
application/xml:
schema:
$ref: '#/components/schemas/Pet'
'400':
description: Invalid ID supplied
'404':
description: Pet not found
delete:
tags:
- pet
summary: Deletes a pet
operationId: deletePet
parameters:
- name: api_key
in: header
required: false
schema:
type: string
- name: petId
in: path
description: Pet id to delete
required: true
schema:
type: integer
format: int64
responses:
'400':
description: Invalid ID supplied
'404':
description: Pet not found
components:
schemas:
Pet:
type: object
required:
- name
- photoUrls
properties:
id:
type: integer
format: int64
name:
type: string
example: doggie
photoUrls:
type: array
xml:
name: photoUrl
wrapped: true
items:
type: string
status:
type: string
description: pet status in the store
enum:
- available
- pending
- sold
requestBodies:
Pet:
content:
application/json:
schema:
$ref: '#/components/schemas/Pet'
description: Pet object that needs to be added to the store
required: true
2️⃣ Import it in Postman.
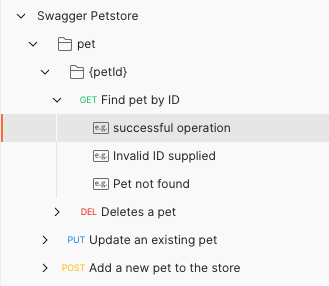
3️⃣ Add examples to your requests.
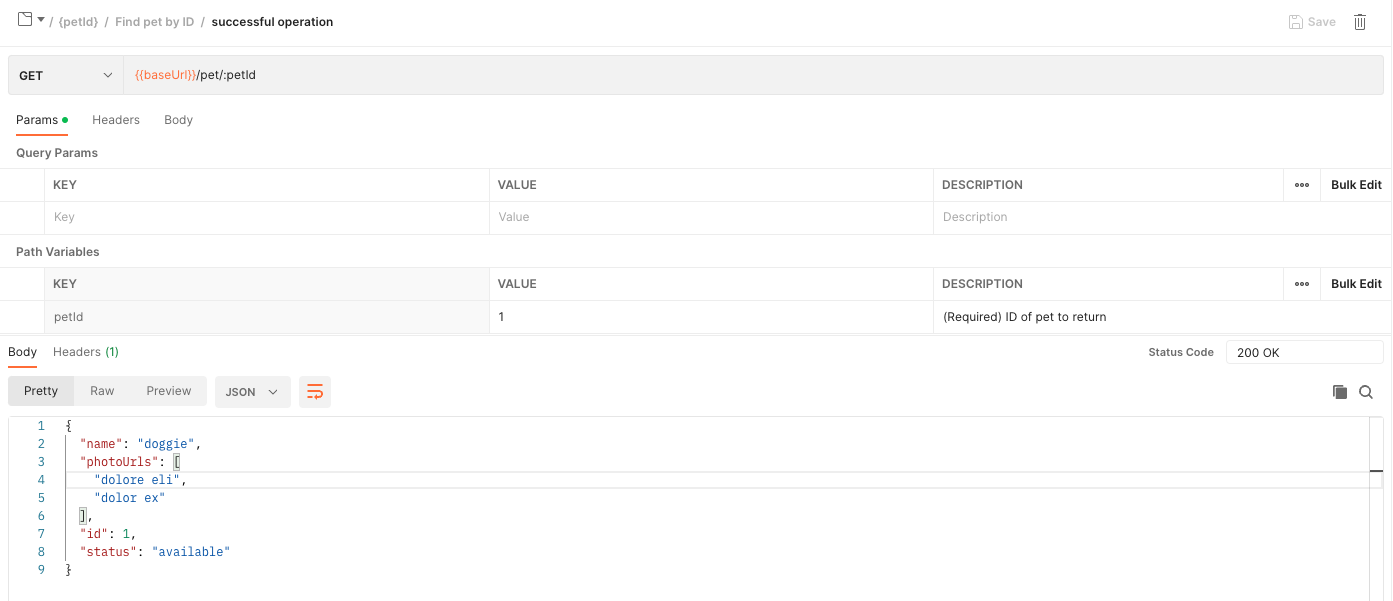
4️⃣ Create a mock server based on the Request Collection
And that's it. Let's now test our Mock Server
.
Get the Mock Server
's URL by clicking on Copy URL
.
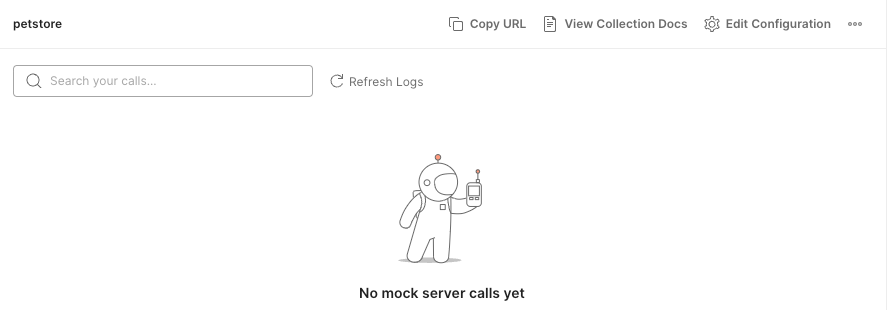
In my case it's: https://4271e5d3-910d-44ce-8ff3-cdf64991c219.mock.pstmn.io
5️⃣ Go to your terminal and send a request:
curl --location --request GET 'https://4271e5d3-910d-44ce-8ff3-cdf64991c219.mock.pstmn.io/pet/1' --header 'Accept: application/json'
