Java Interview Questions (series) - `finalize` method
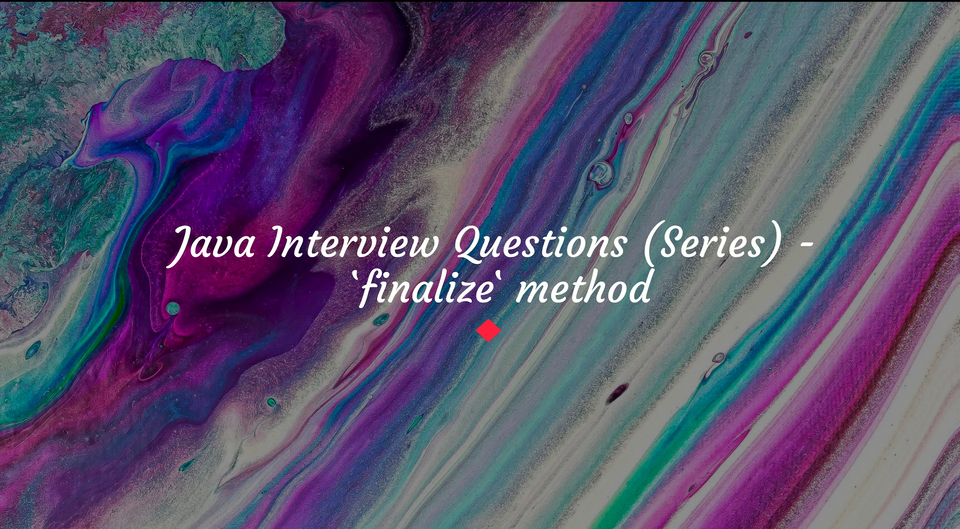
Beware of the F-word! 😈
Brief
The difference between the three Java F-words is one of the interviewers' favourite these days.
In the previous post we covered the finally
keyword.
🚀 Today we'll speak about the finalize
method.
Implementation
The finalize
method is found on the Object
class. That means every class created has access to it.
It's empty so that it can be customizable depending on your particular cases.
public class Object {
. . .
protected void finalize() throws Throwable { }
}
In order to understand how it works and how it's used, we need to take a step back and talk about how the Garbage Collector works in Java.
Garbage Collector
This component looks over the heap memory ( where all the objects are stored by JVM) to find unreferenced objects.
🔵 Examples of unreferenced objects
-
Car car = new Car(); // create a new Car object car = null;
-
Car car1 = new Car(); // create a new Car object Car car2 = new Car(); // create a second Car object car1 = car2; // the first instance is not referenced anymore
Those found will be destroyed and removed from the memory in a process called automatic memory management. As its name says, it's done automatically so there is no need to do it explicitly, as in other programming languages like C++.
Before destroying the object, the JVM checks if it uses resources that should be released. Those resources may be:
🔹 database connections
🔹 network connections
🔹 file resources, etc.
These resources are closed when the Garbage collector calls finalize()
on the object to be deleted.
🔵 Explicit garbage collection
You can also call it explicitly.
public class Car {
public Car() {
System.out.println("Car object created");
}
@Override
protected void finalize() {
System.out.println("Finalize method called");
}
}
public class FinalizeService {
public void finalizeObject() {
Car car = new Car();
car = null; // unreference object
System.gc(); // call the Garbage Collector
}
}
🔸 Output:
Car object created
Finalize method called
Pitfalls
For the most part you shouldn't interfere with the Garbage Collection process.
There are a few reasons why:
🔹 Executing System.gc()
doesn't start the GC process immediately. The JVM implementation and system resources influence the time of start.
🔹 Since the GC process is dependent on the JVM, the same application may run well on a particular system while behaving worse on another.
🔹 If an exception is thrown from the finalize
method, the GC process stops and may leave the object to be removed in an altered state.
🔹 And last, the finalize
method has been deprecated starting with Java 9 and will eventually be removed.
Stay tuned! 🚀